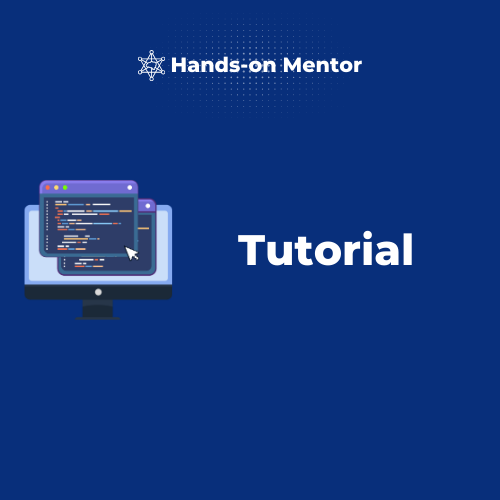
I. Introduction
Python is a powerful programming language that offers various control flow mechanisms to dictate the execution of code. Loops, specifically the while loop and the for loop, play a crucial role in repetitive tasks and data processing in Python. In this tutorial, we will explore the fundamentals of loops, their syntax, and their applications in data science. We will delve into real-world examples and demonstrate how to efficiently iterate through different data structures such as lists, strings, dictionaries, NumPy arrays, and Pandas DataFrames.
II. if-elif-else Construct
The if-elif-else construct allows us to make decisions in our code based on certain conditions. We will understand how the flow of execution changes based on the evaluation of these conditions. Through use cases and practical examples, we will gain a clear understanding of how to employ this construct effectively.
III. while Loop
The while loop is a valuable tool for repetitive tasks, allowing us to execute a block of code repeatedly as long as a specified condition remains true. We will compare it with the if statement and grasp when to choose one over the other. Utilizing a numerical calculation example, we will demonstrate how the while loop can be used to iterate until a particular condition is met.
Example:
# Example: Numerical calculation with a while loop
error = 50
while error > 1:
error /= 4
print(error)
Output:
12.5
3.125
0.78125
IV. for Loop
The for loop is a versatile loop that simplifies iteration over various data structures, including lists and strings. We will explore the syntax and use enumerate() to access both the index and value during iteration. An analogy will help us understand its power in simplifying repetitive tasks.
Example:
# Example: Iterating over a list using for loop and enumerate()
heights = [1.73, 1.68, 1.71, 1.89]
for index, height in enumerate(heights):
print(f"Person {index+1}: Height {height} meters")
Output:
Person 1: Height 1.73 meters
Person 2: Height 1.68 meters
Person 3: Height 1.71 meters
Person 4: Height 1.89 meters
V. Looping Data Structures Part 1
In this section, we will focus on looping over more complex data structures like dictionaries and NumPy arrays. We will see how to efficiently iterate through dictionary key-value pairs using the items() method. Additionally, we will explore the nuances of handling 1D and 2D NumPy arrays using simple for loops and the nditer() function.
Example:
# Example: Iterating over dictionary key-value pairs using items()
world_population = {
'China': 1444216107,
'India': 1393409038,
'United States': 332915073,
'Indonesia': 276361783,
'Pakistan': 225199937
}
for country, population in world_population.items():
print(f"{country}: {population} people")
Output:
China: 1444216107 people
India: 1393409038 people
United States: 332915073 people
Indonesia: 276361783 people
Pakistan: 225199937 people
VI. Looping Data Structures Part 2
This section introduces us to Pandas DataFrames, a powerful data structure used extensively in data science. We will learn how to iterate over DataFrame rows using iterrows() and access specific elements in rows. To enhance efficiency, we will also explore using the apply() function for element-wise operations on DataFrame columns.
Example:
# Example: Iterating over DataFrame rows using iterrows()
import pandas as pd
data = {'Country': ['China', 'India', 'USA'],
'Population': [1444216107, 1393409038, 332915073],
'Capital': ['Beijing', 'New Delhi', 'Washington, D.C.']}
brics_df = pd.DataFrame(data)
for index, row in brics_df.iterrows():
print(f"Country: {row['Country']}, Population: {row['Population']}, Capital: {row['Capital']}")
Output:
Country: China, Population: 1444216107, Capital: Beijing
Country: India, Population: 1393409038, Capital: New Delhi
Country: USA, Population: 332915073, Capital: Washington, D.C.
VII. Conclusion
In this tutorial, we explored the power and versatility of loops in Python, along with their applications in data science. We covered the if-elif-else construct, the while loop, and the for loop. We also learned how to iterate over different data structures, including dictionaries, NumPy arrays, and Pandas DataFrames. Armed with this knowledge, you are now better equipped to handle repetitive tasks and efficiently process data in Python. Keep practicing and exploring, and you will become proficient in using loops for data manipulation and analysis.